- Roblox To Lua C Download
- Roblox Gui To Lua Converter
- Roblox Tobu Candyland Id
- Roblox Tobu Candyland
- Roblox Lua Compiler
High level languages such as Lua, Python or Go lacks the features and compatibility needed to develop Roblox exploits, whereas low level languages such as CC and Ada can manipulate structures that are necessary to make a working hack. Or go to ToolsLua Engine. Paste everything from scriptexecutor.lua into the text field. Hit Execute script.
This style guide aims to unify as much Lua code at Roblox as possible under the same style and conventions.
This guide is designed after Google's C++ Style Guide. Although Lua is a significantly different language, the guide's principles still hold.
Guiding Principles¶
- The purpose of a style guide is to avoid arguments.
- There's no one right answer to how to format code, but consistency is important, so we agree to accept this one, somewhat arbitrary standard so we can spend more time writing code and less time arguing about formatting details in the review.
- Optimize code for reading, not writing.
- You will write your code once. Many people will need to read it, from the reviewers, to any one else that touches the code, to you when you come back to it in six months.
- All else being equal, consider what the diffs might look like. It's much easier to read a diff that doesn't involve moving things between lines. Clean diffs make it easier to get your code reviewed.
- Avoid magic, such as surprising or dangerous Lua features:
- Magical code is really nice to use, until something goes wrong. Then no one knows why it broke or how to fix it.
- Metatables are a good example of a powerful feature that should be used with care.
- Be consistent with idiomatic Lua when appropriate.
File Structure¶
Files should consist of these things (if present) in order:
- An optional block comment talking about why this file exists
- Don't attach the file name, author, or date -- these are things that our version control system can tell us.
- Services used by the file, using
GetService
- Module imports, using
require
- Module-level constants
- Module-level variables and functions
- The object the module returns
- A return statement!
Requires¶
- All
require
calls should be at the top of a file, making dependencies static. Use relative paths when importing modules from the same package.
Use absolute paths when importing modules from a different package.
Metatables¶
Metatables are an incredibly powerful Lua feature that can be used to overload operators, implement prototypical inheritance, and tinker with limited object lifecycle.
At Roblox, we limit use of metatables to a couple cases:
- Implementing prototype-based classes
- Guarding against typos
Prototype-based classes¶
The most popular pattern for classes in Lua is sometimes referred to as the One True Pattern. It defines class members, instance members, and metamethods in the same table and highlights Lua's strengths well.
First up, we create a regular, empty table:
Next, we assign the __index
member on the class back to itself. This is a handy trick that lets us use the class's table as the metatable for instances as well.
When we construct an instance, we'll tell Lua to use our __index
value to find values that are missing in our instances. It's sort of like prototype
in JavaScript, if you're familiar.
In most cases, we create a default constructor for our class. By convention, we usually call it new
.
Methods that don't operate on instances of our class are usually defined using a dot (.
) instead of a colon (:
).
We can also define methods that operate on instances. These are just methods that expect their first argument to be an instance. By convention, we define them using a colon (:
):
At this point, our class is ready to use!
We can construct instances and start tinkering with it:
Further additions you can make to your class as needed:
- Introduce a
__tostring
metamethod to make debugging easier - Define quasi-private members using two underscores as a prefix
Add a method to check type given an instance, like:
Guarding against typos¶
Indexing into a table in Lua gives you nil
if the key isn't present, which can cause errors that are difficult to trace!
Our other major use case for metatables is to prevent certain forms of this problem. For types that act like enums, we can carefully apply an __index
metamethod that throws:
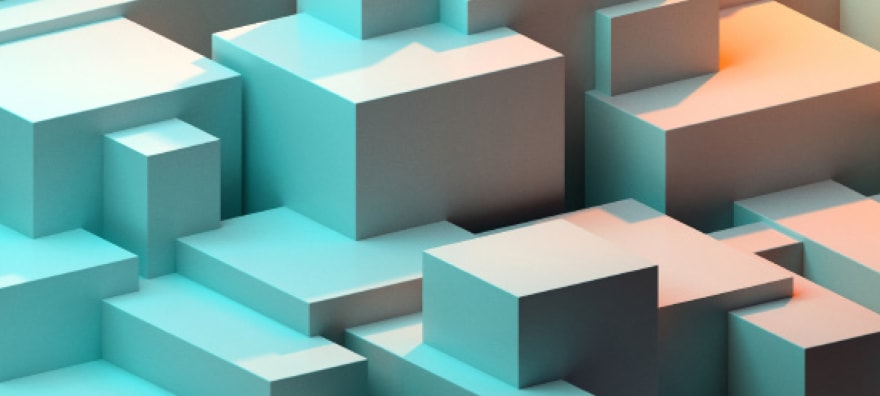
Roblox To Lua C Download
Since __index
is only called when a key is missing in the table, MyEnum.A
and MyEnum.B
will still give you back the expected values, but MyEnum.FROB
will throw, hopefully helping engineers track down bugs more easily.
General Punctuation¶
- Don't use semicolons
;
. They are generally only useful to separate multiple statements on a single line, but you shouldn't be putting multiple statements on a single line anyway.
General Whitespace¶
- Indent with tabs.
- Keep lines under 120 columns wide, assuming four column wide tabs.
- Luacheck will warn for lines over 120 bytes wide; it isn't accurate with tab characters!
- Wrap comments to 80 columns wide, assuming four column wide tabs.
- This is different than normal code; the hope is that short lines help improve readability of comment prose, but is too restrictive for code.
- Don't leave whitespace at the end of lines.
- If your editor has an auto-trimming function, turn it on!
- Add a newline at the end of the file.
No vertical alignment!
- Vertical alignment makes code more difficult to edit and often gets messed up by subsequent editors.
Good:
Bad:
Use a single empty line to express groups when useful. Do not start blocks with a blank line. Excess empty lines harm whole-file readability.
Use one statement per line. Put function bodies on new lines.
Good:
Bad:
This is especially true for functions that return multiple values. Compare these two statements:
It's much easier to spot the mistake (and much harder to make in the first place) if the function isn't on one line.
This is also true for
if
blocks, even if their body is just areturn
statement.Good:
Bad:
Most of the time this pattern is used, it's because we're performing validation of an input or condition. It's much easier to add logging, or expand the conditional, when the statement is broken across multiple lines. It will also diff better in code review.
Put a space before and after operators, except when clarifying precedence.
Good:
Bad:
Put a space after each commas in tables and function calls.
Good:
Bad:
When creating blocks, inline any opening syntax elements.
Good:
Bad:
Avoid putting curly braces for tables on their own line. Doing so harms readability, since it forces the reader to move to another line in an awkward spot in the statement.
Good:
Bad:
Exception:
Newlines in Long Expressions¶
First, try and break up the expression so that no one part is long enough to need newlines. This isn't always the right answer, as keeping an expression together is sometimes more readable than trying to parse how several small expressions relate, but it's worth pausing to consider which case you're in.
It is often worth breaking up tables and arrays with more than two or three keys, or with nested sub-tables, even if it doesn't exceed the line length limit. Shorter, simpler tables can stay on one line though.
Prefer adding the extra trailing comma to the elements within a multiline table or array. This makes it easier to add new items or rearrange existing items.
Break dictionary-like tables with more than a couple keys onto multiple lines.
Good:
Bad:
Break list-like tables onto multiple lines however it makes sense.
- Make sure to follow the line length limit!
For long argument lists or longer, nested tables, prefer to expand all the subtables. This makes for the cleanest diffs as further changes are made.
In some situations where we only ever expect table literals, the following is acceptable, though there's a chance automated tooling could change this later. In particular, this comes up a lot in Roact code (doSomething
being Roact.createElement
).
However, this case is less acceptable if there are any non-tables added to the mix. In this case, you should use the style above.
For long expressions try and add newlines between logical subunits. If you're adding up lots of terms, place each term on its own line. If you have parenthesized subexpressions, put each subexpression on a newline.
- Place the operator at the beginning of the new line. This makes it clearer at a glance that this is a continuation of the previous line.
- If you have to need to add newlines within a parenthesized subexpression, reconsider if you can't use temporary variables. If you still can't, add a new level of indentation for the parts of the statement inside the open parentheses much like you would with nested tables.
- Don't put extra parentheses around the whole expression. This is necessary in Python, but Lua doesn't need anything special to indicate multiline expressions.
For long conditions in
if
statements, put the condition in its own indented section and place thethen
on its own line to separate the condition from the body of theif
block. Break up the condition as any other long expression.Good:
Bad:
Blocks¶
Don't use parentheses around the conditions in
if
,while
, orrepeat
blocks. They aren't necessary in Lua!Use
do
blocks if limiting the scope of a variable is useful.
Literals¶
Use double quotes when declaring string literals.
- Using single quotes means we have to escape apostrophes, which are often useful in English words.
- Empty strings are easier to identify with double quotes, because in some fonts two single quotes might look like a single double quote (
'
vs'
)
Good:
Bad:
- Single quotes are acceptable if the string contains double quotes to reduce escape sequences.
Exception:
- If the string contains both single and double quotes, prefer double quotes on the outside, but use your best judgement.
Tables¶
- Avoid tables with both list-like and dictionary-like keys.
- Iterating over these mixed tables is troublesome.
- Iterate over list-like tables with
ipairs
and dictionary-like tables withpairs
.- This helps clarify what kind of table we're expecting in a given block of code!
Add trailing commas in multi-line tables.
- This lets us re-sort lines with a single keypress and makes diffs cleaner when adding new items.
Functions¶
- Keep the number of arguments to a given function small, preferably 1 or 2.
Always use parentheses when calling a function. Lua allows you to skip them in many cases, but the results are typically much harder to parse.
Good:
Bad:
Of particular note, the last example - using the curly braces as if they were function call syntax - is common in other Lua codebases, but while it's more readable than other ways of using this feature, for consistency we don't use it in our codebase.
Declare named functions using function-prefix syntax. Non-member functions should always be local.
Good:
Bad:
Exception:
When declaring a function inside a table, use function-prefix syntax. Differentiate between
.
and:
to denote intended calling convention.Good:
Bad:
- Wrap comments to 80 columns wide.
- It's easier to read comments with shorter lines, but fitting code into 80 columns can be challenging.
Use single line comments for inline notes:
- If the comment spans multiple lines, use multiple single-line comments.
- Sublime Text has an automatic wrap feature (alt+Q on Windows) to help with this!
Use block comments for documenting items:
- Use a block comment at the top of files to describe their purpose.
- Use a block comment before functions or objects to describe their intent.
Comments should focus on why code is written a certain way instead of what the code is doing.
Good:
Bad:
No section comments.
Comments that only exist to break up a large file are a code smell; you probably need to find some way to make your file smaller instead of working around that problem with section comments. Comments that only exist to demark already obvious groupings of code (e.g.
--- VARIABLES ---
) and overly stylized comments can actually make the code harder to read, not easier. Additionally, when writing section headers, you (and anyone else editing the file later) have to be thorough to avoid confusing the reader with questions of where sections end.Some examples of ways of breaking up files:
- Move inner classes and static functions into their own files, which aren't included in the public API. This also makes testing those classes and functions easier.
- Check if there are any existing libraries that can simplify your code. If you're writing something and think that you could make part of this into a library, there's a good chance someone already has.
If you can't break the file up, and still feel like you need section headings, consider these alternatives.
If you want to put a section header on a group of functions, put that information in a block comment attached to the first function in that section. You should still make sure the comment is about the function its attached to, but it can also include information about the section as a whole. Try and write the comment in a way that makes it clear what's included in the section.
The same can be done for a group of variables in some cases. All the same caveats apply though, and you have to consider whether one block comment or a normal comment on each variable (or even using just whitespace to separate groups) would be more readable.
- General organization of your code can aid readibility while making logical sections more obvious as well. Module level variables and functions can appear in any order, so you can sometimes put a group of variables above a group of functions to make a section.
Naming¶
- Spell out words fully! Abbreviations generally make code easier to write, but harder to read.
- Use
PascalCase
names for class and enum-like objects. - Use
PascalCase
for all Roblox APIs.camelCase
APIs are mostly deprecated, but still work for now. - Use
camelCase
names for local variables, member values, and functions. - For acronyms within names, don't capitalize the whole thing. For example,
aJsonVariable
orMakeHttpCall
. - The exception to this is when the abbreviation represents a set. For example, in
anRGBValue
orGetXYZ
. In these cases,RGB
should be treated as an abbreviation ofRedGreenBlue
and not as an acronym. - Use
LOUD_SNAKE_CASE
names for local consants. - Prefix private members with an underscore, like
_camelCase
.- Lua does not have visibility rules, but using a character like an underscore helps make private access stand out.
- A File's name should match the name of the object it exports.
- If your module exports a single function named
doSomething
, the file should be nameddoSomething.lua
.
- If your module exports a single function named
FooThing.lua
:
Yielding¶
Do not call yielding functions on the main task. Wrap them in coroutine.wrap
or delay
, and consider exposing a Promise or Promise-like async interface for your own functions.
Pros:
- Roblox's yielding model makes calling asynchronous tasks transparent to the user, which lets users call complicated functions without understanding coroutines or other async primitives.
Cons:
Unintended yielding can cause hard-to-track data races. Simple code involving callbacks can cause confusing bugs if the input callback yields.
Error Handling¶
When writing functions that can fail, return success, result
, use a Result
type, or use an async primitive that encodes failure, like Promise
.
Do not throw errors except when validating correct usage of a function.
Pros:
- Using exceptions lets unhandled errors bubble up 'automatically' to your caller
- Stack traces are automatically attached to errors

Cons:
- Lua can only throw strings as errors, which makes distinguishing between them very difficult
- Exceptions are not encoded into a function's contract explicitly. By returning
success, result
, you force your caller to consider whether an error will happen.
Exceptions:
- When calling functions that communicate failure by throwing, wrap calls in
pcall
and make it clear via comment what kinds of errors you're expecting to handle.
General Roblox Best Pratices¶
- All services should be referenced using
game:GetService
at the top of the file. - When importing a module, use the name of the module for its variable name.
Synapse X is the leading exploit script executor tool for Roblox. Our software can bypass any anti-cheat and inject scripts at a 3x faster rate than the industry standard.
Undetected
You can feel safe using Synapse X with Roblox. Our hack is undetected, which means you can’t get banned. Use as many scripts as you want!
ALL PLATFORMS
We’ve come a long way with our exploit tool. You can now use Synapse X with any game platform, including PS4, PC, Mobile and Xbox.
25k+ users
Our Roblox hack is trusted and used by over 25,000 users and counting. Synapse X really works, you can count on that. Join the other users now!
What’s Synapse X And How Does It Work?
Synapse X is a software that you use to inject scripts into Roblox. In order to use scripts in Roblox, you need to inject them into the game. This is what Synapse X does so well.
Simply download our tool, and start injecting scripts into Roblox!
There’s no risk of getting banned in-game, since Synapse X is completely undetected.
Roblox Gui To Lua Converter
Next-Generation Script Executor
A script executor is a medium that allows you to implement scripts in a different scripting language. There is a common misconception that a scripting language is similar to a programming language. Although the line between them is quite thin, they are different. It is vital to remember this to get the best from every scripting quest you undertake.
One of their major differences is that a scripting language is built on a platform that allows a code to be written with automation. On the other hand, programming languages rely mostly on traditional code writing, where a programmer writes them by hand before they are executed.

At Synapse X, this is the service we offer. We allow our users to make and implement scrips for various programs through our impressive Synapse Lua (Slua) engine. We will guide you through everything you need to know about a scripting engine, how to use it and why Synapse X is the best and most reliable engine you could use.
To efficiently implement our scripting engine, you must first understand what a scripting language, how to use it, and how it all comes together. A scripting language will differ based on the scripting language it supports. They tend to run on smaller programs and, at times, will sidestep a compiler.
They give programmers access to the executable code or the source code, and in the compiled language, it might become inaccessible. Our scripting engine, Slua, is compatible with many existing software architectures, making it very reliable. You thus won’t have to go through many steps to get proper results and one that will be executed easily.
Injection for Roblox
Our scripting engine is mainly used for Roblox game. This is an online game creation platform that Roblox Corporation developed in 2006. On the platform, you can develop games or play games developed by other programmers. This gives you a new gaming perspective every time you run the platforms.
You get to play a wide range of games, all of which are different. It also allows you to explore the creativity of other players and let them see your own too. With the help of our Synapse Lua scripting engine, you can easily and conveniently create and run scripts.
Roblox Tobu Candyland Id
Features
Synapse X offers some of the best and most reliable features that any programmer creating scripts for Roblox would need.
Roblox Tobu Candyland
One of the greatest features that sets us apart from many other scripting engines is speed. It is very responsive, and you won’t have to spend a lot of time waiting for results. This also ensures you can create the perfect scripts for Roblox.
Our scripting engine is also very stable. You won’t experience any crashes while using the bot, as experienced with some scripting engines. This allows all your scripts to run efficiently and reliably. You are thus assured of the best experience with this engine.
Roblox Lua Compiler
Another key feature of our Synapse Lua engine is its compatibility with scripts. It will run all scripts, even those created from different scripting engines such as Java, efficiently. This makes it very convenient and reliable since different users prefer scripting on different engines. Having an engine that can easily run all these scripts is very impressive and reliable.